💸 Merchant Payouts
Merchant initiated payouts to off-chain users
Coinflow makes it possible to payout users without them having to have a wallet setup. The merchant can use their established identifiers to setup the recipient users on the platform. The users will still need to be KYC validated and setup a bank and/or debit card for the distribution.
The workflow is very straightforward:
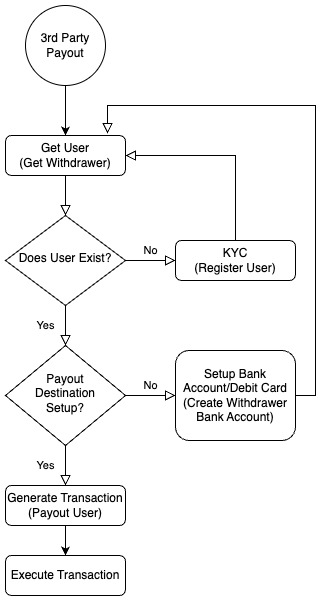
API Headers
For all 3rd Party payout requests, the following headers need to be in the request:
Header | Value |
---|---|
Authorization | All calls in this flow will require your merchant API key which can be created on the dashboard here. |
x-coinflow-auth-user-id | A unique identifier for the user created by you. This is a string which uniquely identifies the user within your system, or if all your users have wallets, the users wallet address. You will register, lookup, add accounts, and generate payout transactions all referencing the user id of a particular user by the user id you submit when registering them. |
User Setup
KYC Users
Users must be KYC validated before anything else can take place. Here is the API endpoint needed:
Payout Destination
A destination for a payout needs to be established, so a bank account or a debit card needs to be entered.
Bank Account
Coinflow accepts all major banks facilitated by Plaid. Here is the API endpoint to setup a bank account:
Debit Card
Coinflow will disburse to eligible debit cards. Here is the API endpoint to setup a debit card:
IBAN Account
For European users, Coinflow accepts IBAN accounts. Here is the API endpoint for an IBAN account:
Push to Card
With push to card, users can offramp directly to a debit card account. Users don't need to take any action to receive the funds other than having a valid Visa/Mastercard debit card linked to their account.
ACH
Regular ACH withdrawals are not instant and take 3 business days to settle.
Same day ACH
Same Day ACH withdrawals allows funds to be sent to a user's bank account on the same day that the transaction is initiated.
Generate Payout Transaction
Once KYC has occurred and a payout destination setup, the payout transaction can be created. Here is the API endpoint to generate the transaction:
Note: This endpoint requires merchant authorization (admin scope) and not the aforementioned headers.
Example
The example assumes that the user has been KYC'd and a bank account has been setup.
const axios = require('axios');
const options = {
method: 'GET',
url: 'https://api-sandbox.coinflow.cash/api/withdraw',
headers: {
accept: 'application/json',
'x-coinflow-auth-user-id': '<unique-user-id>',
'Authorization': '<Merchant API Key>'
}
};
axios
.request(options)
.then(function (response) {
const token = response.data.withdrawer.bankAccounts[0].token;
generate(token);
})
.catch(function (error) {
console.error(error);
});
function generate(token) {
const options = {
method: 'POST',
url: 'https://api-sandbox.coinflow.cash/api/merchant/withdraws/payout',
headers: {
accept: 'application/json',
'content-type': 'application/json',
Authorization: '<API-key>'
},
data: {
blockchain: 'solana',
amount: {cents: 500},
speed: 'standard',
wallet: '<merchant-wallet>',
userId: '<unique-user-id>',
account: token
}
};
axios
.request(options)
.then(function (response) {
console.log('Transaction: ' + response.data.transactions[0]);
})
.catch(function (error) {
console.error(error);
});
}
Updated 4 months ago