🪝Webhooks
Webhooks are available for the lifecycle of KYC and Withdrawals
Webhooks allow you to subscribe to events from Coinflow about the lifecycle of the KYC/KYB process and withdrawals.
Setup
- To set up Webhooks for your application go to the Coinflow Admin Dashboard > Webhooks.
- Copy your Webhook Validation Key.
Keep this Validation Key secret, but if it gets leaked you can easily regenerate it.
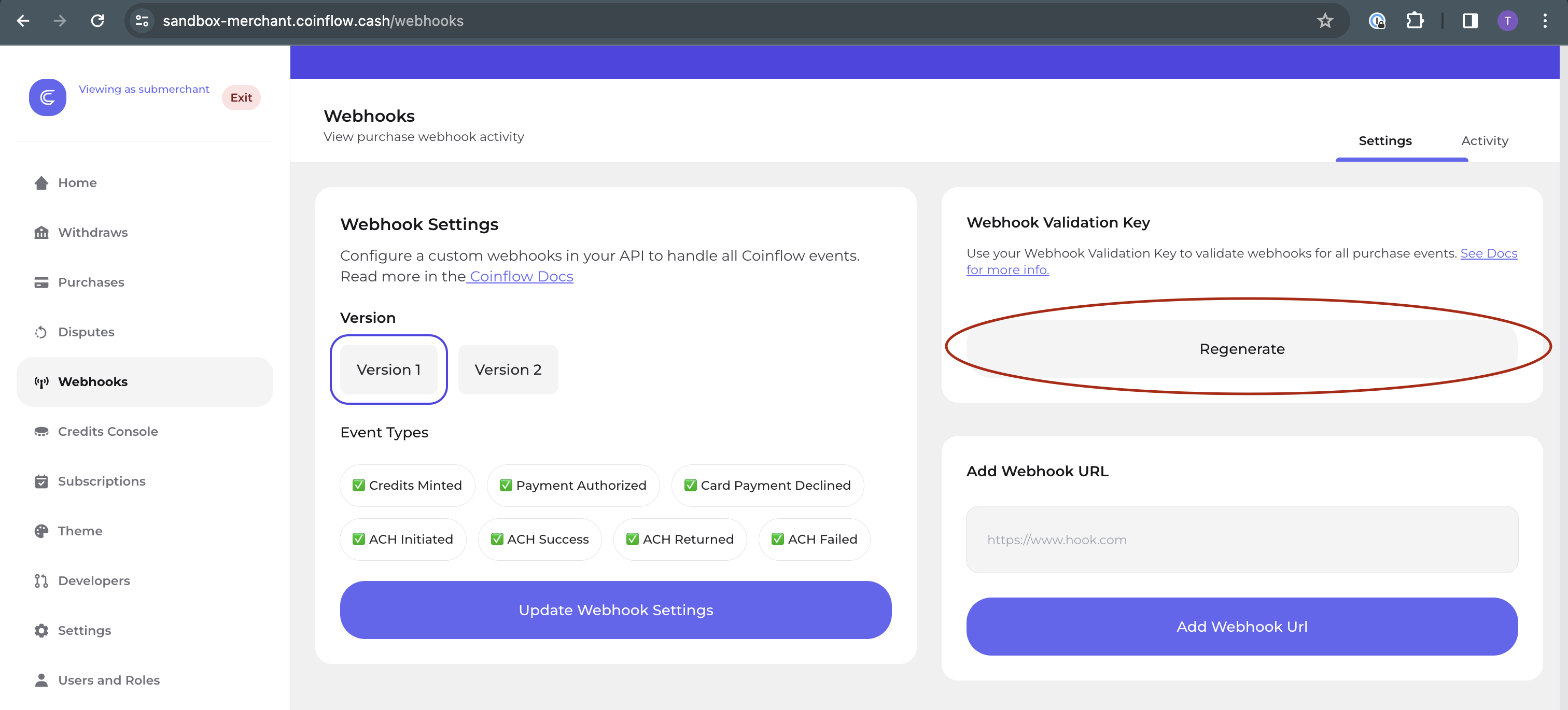
- Enter your webhook settings configuration. All of the relevant event types prefixed with
KYC
orWithdraw
:
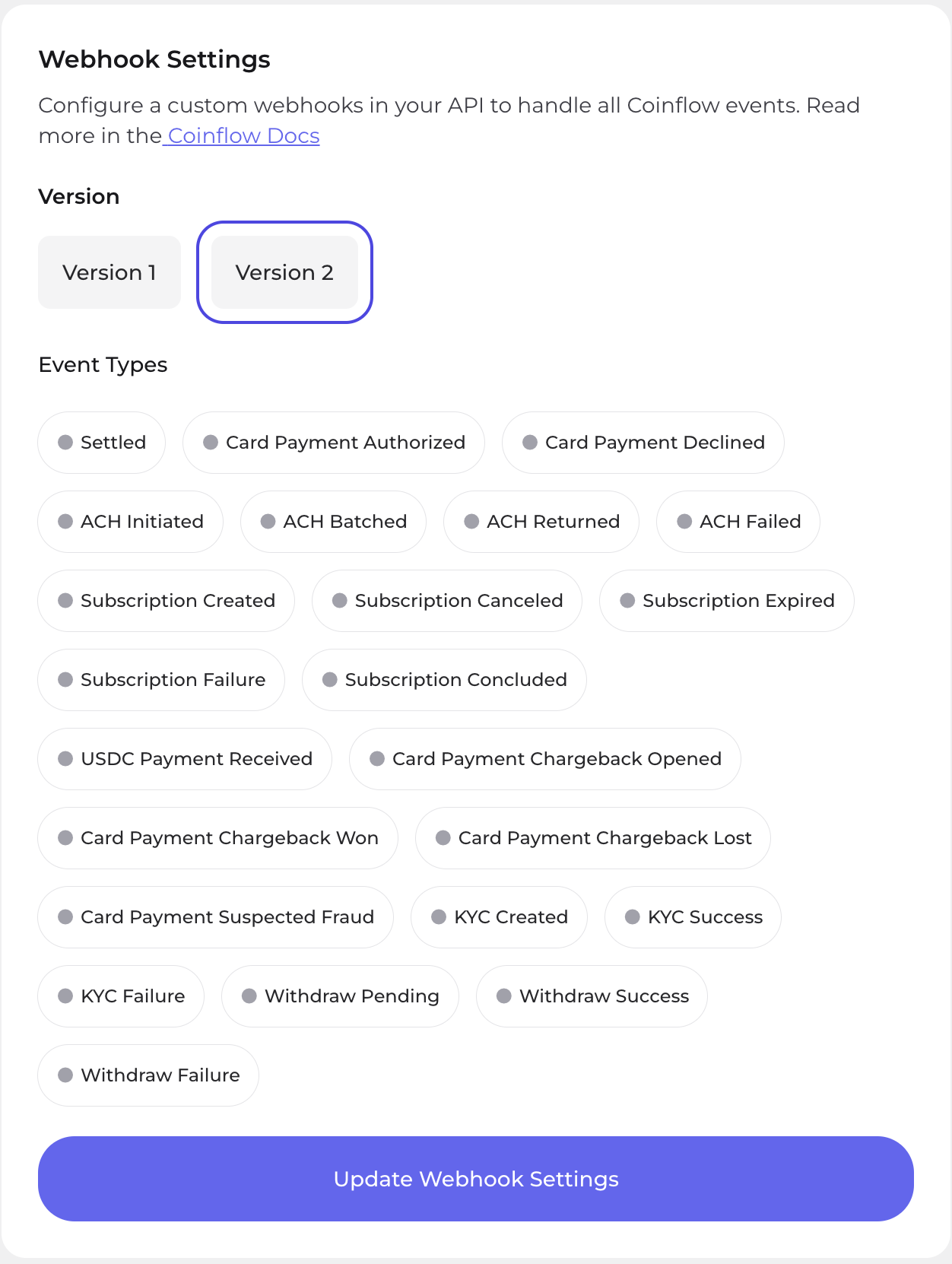
- Enter a URL route to your server endpoint
- KYC and Withdraw event types are only available in Version 2
- Select the event types that should post notification (see addendum for event type detail)
- In your server create a route that accepts a POST request on that url and route
Make sure the
Authorization
header in this request is equal to your Validation Key to validate that this request is coming from Coinflow.
router.post('/withdraw-webhook', async (req, res) => {
try {
const {data} = req.body;
const {signature, wallet, total} = data;
console.log(`Coinflow withdraw (${signature}) for ${wallet}`);
const authHeader = req.get('Authorization');
// Authorize using your Validation key
if (authHeader !== process.env.COINFLOW_VALIDATION_KEY)
throw new ControllerError('User not allowed', 401);
else handleEvent() // react to event
res.sendStatus(200);
} catch (e) {
handleError(res, e);
}
});
Webhooks will retry until your server returns a 200 response code
or until it times out after 36 hours. Webhooks will also time out after 5 seconds without a response and will retry, so make sure your server responds within 5 seconds.
- The webhooks will contain the following data:
KYC
{
eventType: string,
category: 'KYC',
created: string,
data: {
wallet: string,
blockchain: string,
email: string,
},
}
Withdraw
{
eventType: string,
category: 'Withdraw',
created: string,
data: {
wallet: string,
blockchain: string,
signature: string,
userFees: {
cents: number,
},
userGasFees: {
cents: number,
},
merchantFees: {
cents: number,
},
merchantGasFees: {
cents: number,
},
total: {
cents: number,
},
currency: string,
},
}
- Done! Try listening to different event types by subscribing to the different options below
KYC Event Types
Event Type | Description |
---|---|
KYC Created | The KYC/KYB process has been initiated |
KYC Success | The KYC/KYB submission passed |
KYC Failure | The KYC/KYB submission failed |
Withdraw Event Types
Event Type | Description |
---|---|
Withdraw Pending | A withdrawal has been initiated |
Withdraw Success | The withdrawal is valid and submitted to the bank for settlement |
Withdraw Failure | The withdrawal failed |
Updated 5 days ago